1. Data Types, Operations, and Variables
One way to understand programming is as a process of transforming data. A simple calculator performing addition is programmed, for example, to transform two numbers into one.
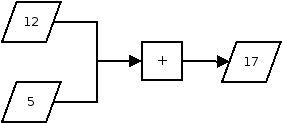
It is therefore necessary to consider,
- Types of data
- Operations on data
- Naming of data
Data Types and Their Operations
There are many built-in data types in python1. For now, we need only focus on the following,
- Integers (
int
) - Strings (
str
) - Booleans (
bool
)
Integers
Integers represent whole numbers, such as 0, 12, 93214, -1, and so on. Try the following, and observe the output,
>>> 1
>>> 52
>>> 0
>>> -3
>>> 2 + 6
>>> 2 - 6
>>> 2 * 6
>>> 2 ** 6
>>> 2 / 6
>>> 2 // 6
>>> 2 % 6
>>> (2 + 6) * 3 / 10
>>> 2 / 0
>>> 2 // 0
As you may have noticed some familiar operators, such as addition (+
), subtraction (-
), multiplication (*
), and division (/
). The double asterisks (**
) is the 'power' operator, which raises the first number to the power of the second; the double forward slashes (//
) is the 'floored division' operator, which divides the first number by the second and gives the answer rounded down. The percent sign (%
) is the modulo operator, which gives the remainder of the division of the first number by the second.2 The order of operations apply.
The last two expressions raises an error, as division by zero is not allowed.
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ZeroDivisionError: division by zero
Errors in programming are extremely common, but generally harmless. Don't be afraid to run a command and fail!
Strings
Strings are sequences of characters, which can include letters, numbers, and symbols. Strings must be surrounded by either single quotes ('
) or double quotes ("
).3 Try the following examples:
>>> 'abcg'
>>> '!@#$%&'
>>> '12347'
>>> 'abc123'
>>> 'This is a sentence'
>>> 'You can use single quotes'
>>> "or double quotes"
>>> 'as long as you use the same ones for each string...'
>>> "There can be 4 mix 0f numbers, and symb*$s!"
If you find the IDLE spitting errors, for example,
>>> 'This is a sentence
File "<stdin>", line 1
'This is a sentence
^
SyntaxError: EOL while scanning string literal
Make sure that you have surrounded both ends of the string with quotation marks, and that for each string you have used the same type of quotation marks.
Do not confuse an integer with a string. The integer 12
is not the same as the string '12'
. Integers are representations of a numerical value, while strings are just characters. The integer 12
represents the quantity 12
. The string '12'
represents the character '1'
followed by the character '2'
. Superficially, the former is not wrapped with quotation marks, but the latter is.4
Like integers, strings can be operated upon. To concatenate5 strings, we use the plus (+
) operator.
>>> 'a' + 'b'
>>> 'a' + 'b' + 'c' + '1' + '!'
>>> 'hello, ' + 'how are you today' + '?'
Booleans
Unlike integers and strings, which may take up any number of values6, booleans can only be True
or False
.
>>> True
>>> False
As we will see shortly, booleans are absolutely essential in programming. There are three boolean operators which come in the form of words rather than symbols: not
, and
, or
.
>>> not True
>>> not False
>>> False and False
>>> True and False
>>> True and True
>>> False or False
>>> True or False
>>> True or True
>>> not (not True or (False and not True))
-
I use the term 'number' here, instead of integer, as these operations can also apply to other data types, including other representations of numbers. ↩
-
Which type of quotation marks you use depends on your preference, and sometimes the context. Here is a decent forum post on this issue. ↩
-
Don't worry if this doesn't yet make sense to you. It's may be rather counter-intuitive at first. ↩
-
To connect or combine ↩
-
...which may virtually take up any number of values... ↩