6. Sending Messages
Creating a Bot Account
First, we need to have a telegram account (bot) to access. Talk to @Botfather, a telegram bot by telegram to create telegram bots, to create a bot. Copy and paste the HTTP API somewhere.1
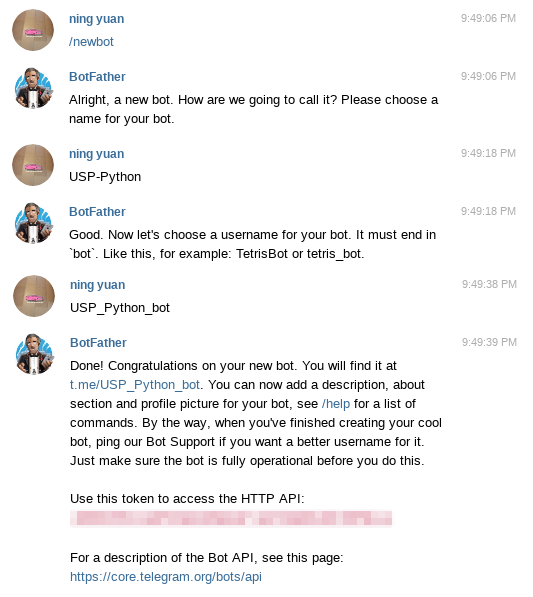
You will also need to know your own telegram user ID, so the bot knows who to send messages to. Talk to @userinfobot to get this information. Once again, copy this information down somewhere.
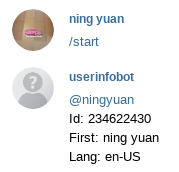
Finally, before we start writing code, we have to /start
our bot. Open up a private message with your bot by searching its username, then hit the start button. Don't worry, nothing's supposed to happen.
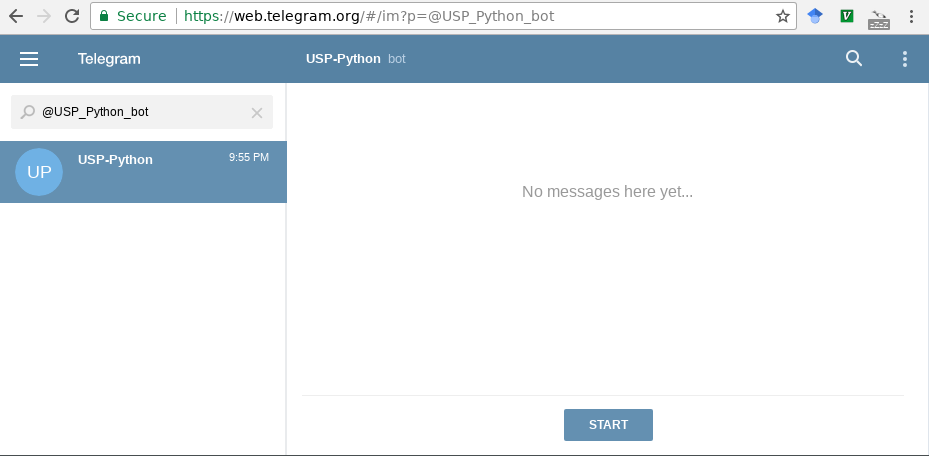
Sending a Message, Part 1
Now, let's write our program. In the first few lines, we need to import the telegram library, and store our HTTP API and user ID in some variables.
import telegram
api_key = '<your api key here>'
user_id = '<your user id here>'
Now, we need to store a representation of the telegram bot into a variable. After this, we will be able to use that variable to tell our bot what to do. Use the Bot
method of the telegram
library like so, passing the HTTP API api_key
as argument2.
bot = telegram.Bot(token=api_key)
Finally, we are ready to send a message from our bot. Use the send_message
method, with appropriate arguments to tell the bot where to send the message to (chat_id
), and what to say (text
).
bot.send_message(chat_id=user_id, text='USP-Python has started up!')
Save your file, and run the program.
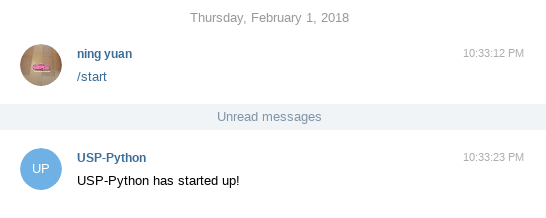
Don't be intimidated by these methods! They work like functions do:
- They have a name, such as
telegram.Bot
, orbot.send_message
3 - They have rounded brackets right after their name...
- and within these brackets are arguments such as
chat_id=user_id
The only difference is that we must use the argument_name=argument_value
notation.4
Checkpoint
If your program doesn't seem to work, check that it looks something like this:
import telegram
api_key = '<your api key here>'
user_id = '<your user id here>'
bot = telegram.Bot(token=api_key)
bot.send_message(chat_id=user_id, text='USP-Python has started up!')
Take note of the error message, particularly which line the error originates from. Then, compare that line in your program to a matching line above. Are there any differences? If so, what are the differences exactly? Amend your program so the lines match. Did that fix the error? If it did, why?
If your program works, leave it as it is---it doesn't have to be an exact match!
Questions & Exercises
- What is the type of
api_key
anduser_id
?
They are strings. - Modify your program so that it sends a second message of your liking.
- Modify your program so that it sends a message to someone else.
-
And keep it private! This is analogous to a password---anyone with this string of characters can access your bot. ↩
-
Methods are similar to functions. We are in fact using the
Bot
method (function) to construct aBot
object, which we store in the variablebot
. ThisBot
object further has methods which will allow us to do things like send messages, photos, videos... ↩ -
Or, the
Bot
method of the moduletelegram
, andsend_message
of the objectbot
, if you want to be technically precise. ↩ -
We have been using positional arguments. These are keyword arguments. Read more? ↩